In modern times, microservices have emerged as a powerful architecture that promotes scalability, flexibility, and efficient management of complex systems. Before, developers always had to suffer from slow deployments and debugging nightmares that led to overall restricted growth of businesses.
In fact, an insightful analysis done by Statista stated that microservices were utilized by 85% of the companies with over 2000+ workers in 2021. Another report published by IBM market development and insights research asserted that companies achieved 30% greater customer retention, 28% improved app performance, 26% increased staff productivity, 29% better customer data security, and faster time-to-market just with a simple switch to Python microservices architecture. Now is it really revolutionary? Let’s find out
In this blog, you’ll understand microservices architecture, their importance over monolithic architecture, and how Python microservices can offer scalable & maintainable systems.
What are Microservices architecture?
Microservices is a super smart architectural approach where complex applications are broken into small and self-contained services for software and product development. These communicate with each other through the APIs aka Application Programming Interfaces. Unlike traditional monolithic applications, dedicated Python developers can build, deploy, and scale businesses independently with the help of loosely coupled microservices. It means each architecture can be individually managed which simplifies maintenance and updates.
Through microservices, you can empower scaling individual high-traffic components instead of entirely depending on demand( as in monolithic architecture). Along with that the easy fault management and feature updates are like the cherry on top helping you grow. To grasp more differences between microservices architecture vs monolithic architecture read along.
Microservices architecture vs monolithic architecture
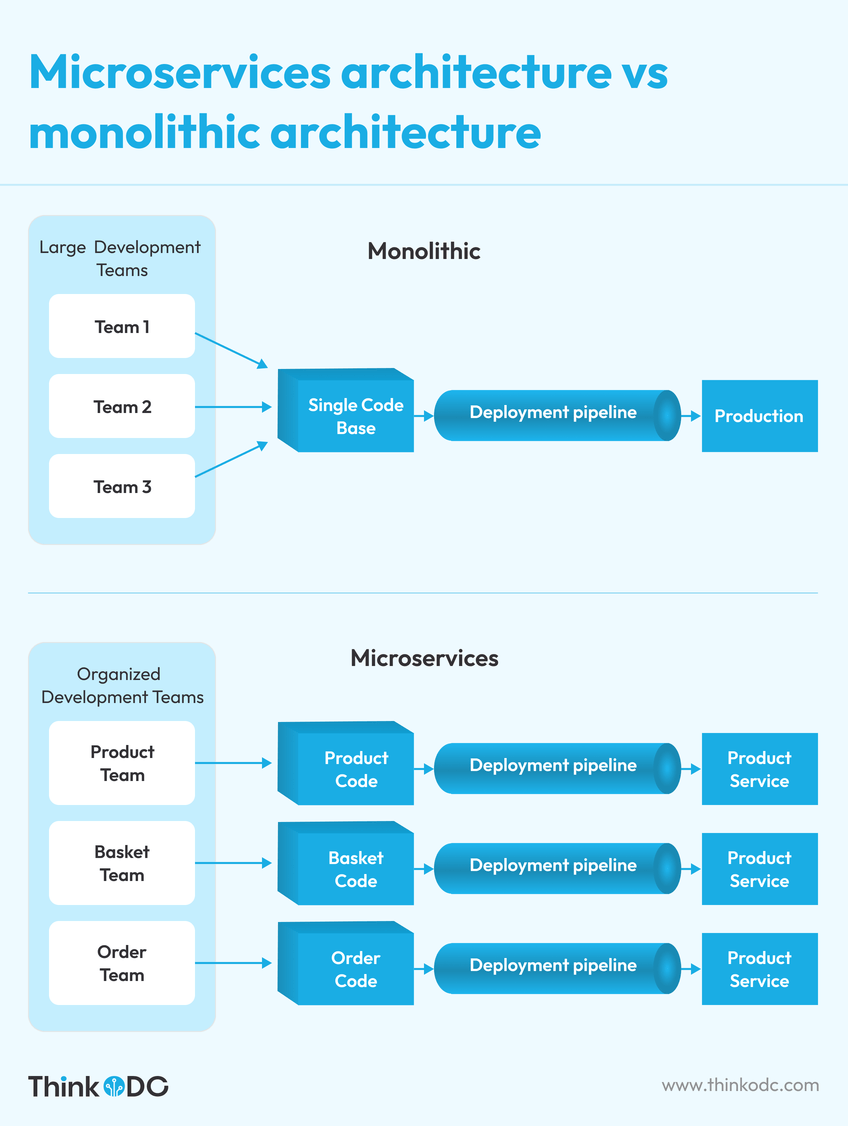
1. Structure and modularity
Microservices: They are composed of small, independent services with specific functions, communicable through APIs.
Monolithic: Built as a single, unified codebase powered with interconnected components.
2. Scalability
Microservices: They can scale individual services independently.
Monolithic: These can scale the entire application as a whole.
3. Development and deployment
Microservices: These architectures support independent development, testing, and deployment, encouraging faster release cycles.
Monolithic: These architectures require rebuilding and redeploying the entire system for any change.
4. Fault isolation
Microservices: Isolates faults and failures in one service without affecting the entire system.
Monolithic: A failure in one part will affect the whole application.
5. Technology stack
Microservices: Allows the use of different technologies for different services.
Monolithic: Utilizes only a single technology stack for the entire application.
With a precise understanding of how microservices architecture gives businesses an upper hand along with flexible approaches. Let’s have a look at the construction of microservices with Python.
How to build microservices with Python?
Before you get bombarded with too much coding jargon here are some prerequisites to understand this guide to building microservices with Python, make sure you have:
- Familiarity with Flask framework.
- Python and Docker should be installed on your system.
No stress if you aren’t familiar! You can hire our top offshore Python developers to build a secure Python web app personalized to your demand.

Tools and frameworks necessary for building microservices in Python
There are several frameworks and libraries that can help build and deploy microservices using Python as follows:
Flask: An easy web framework for building APIs.
FastAPI: It's a high-performance web framework for building APIs.
Docker: A tool for developing, shipping, and running applications in containers.
Kubernetes: An open-source system that helps in the automation of deployment, scaling, and management of containerized applications.
RabbitMQ: A message carrier for communication between microservices.
Consul: Important tool for service discovery and configuration.
Step-by-step guide to building and deploying microservices
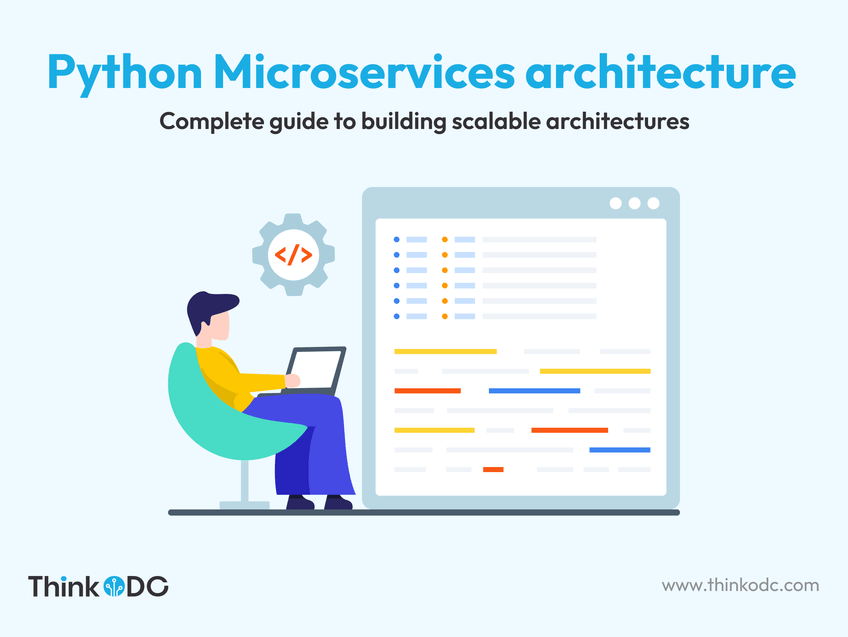
Step 1: Setting up the environment
Confirming the necessary libraries installation on your system, you can set up your Python environment with the following commands:
pip install flask fastapi uvicorn docker
Step 2: Building a microservice with Flask
Let’s create a simple microservice for managing a list of laptops using Flask.
# laptops_service.py
from flask import Flask, request, jsonify
app = Flask(__name__)
laptops = [
{"id": 1, "title": "Lenovo", "model": "S3"},
{"id": 2, "title": "Dell", "model": "Latitude 3.0"}
]
@app.route('/laptops', methods=['GET'])
def get_laptops():
return jsonify(laptops)
@app.route('/laptops', methods=['POST'])
def add_laptop():
new_laptop = request.json
laptops.append(new_laptop)
return jsonify(new_laptop), 201
@app.route('/laptops/<int:book_id>', methods=['DELETE'])
def delete_laptop(laptop_id):
global laptops
laptops = [laptop for laptop in lapto if laptop['id'] != laptop_id]
return '', 204
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
- Flask: Used to create a simple web server.
- laptops: A list to store laptop records.
- @app.route(): Defines the routes for the API endpoints.
- get_laptops(): Returns the list of laptops.
- add_laptop(): Adds a new laptop to the list.
- delete_laptop(): Deletes a laptop by its ID.
Step 3: Building a microservice with FastAPI
Next, let's create another microservice for managing the laptop’s charging port using FastAPI.
# port_service.py
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
from typing import List
app = FastAPI()
class Port(BaseModel):
id: int
name: str
port = [
{"id": 1, "name": "USB-C"},
{"id": 2, "name": "USB-A"}
]
@app.get("/port", response_model=List[Port])
def get_port():
return port
@app.post("/port", response_model=Author, status_code=201)
def add_author(author: Author):
port.append(author.dict())
return author
@app.delete("/port/{author_id}", status_code=204)
def delete_author(author_id: int):
global port
port = [author for author in port if port['id'] != port_id]
return None
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, host='0.0.0.0', port=5001)
-
FastAPI: Used to create a high-performance web server.
-
Port: A data schema to define the structure of port data.
-
port: A list to store charging port records.
-
@app.get(): Defines the GET route to retrieve the port.
-
@app.post(): Defines the POST route to add a new port.
-
@app.delete(): Defines the DELETE route to remove a port by ID.
-
uvicorn: An ASGI server to run the FastAPI application.
Step 4: Containerizing the microservices with Docker
Now to deploy these microservices, we will containerize them using Docker.
First Create a Dockerfile for the laptop service:
# Dockerfile for laptops_service
FROM python:3.9-slim
WORKDIR /app
COPY laptops_service.py /app
RUN pip install flask
CMD ["python", "laptops_service.py"]
Create a Dockerfile for the port service:
# Dockerfile for port_service
FROM python:3.9-slim
WORKDIR /app
COPY port_service.py /app
RUN pip install fastapi uvicorn
CMD ["uvicorn", "port_service:app", "--host", "0.0.0.0", "--port", "5001"]
Build and run the Docker containers:
# Build Docker images
docker build -t laptops-service -f Dockerfile. laptops.
docker build -t port-service -f Dockerfile. port.
# Run Docker containers
docker run -d -p 5000:5000 laptops-service
docker run -d -p 5001:5001 port-service
- FROM python:3.9-slim: Specifies the base image.
- WORKDIR /app: Sets the working directory.
- COPY: Copies the application files to the container.
- RUN pip install: Installs the required libraries.
- CMD: Differentiates the command to run the application.
Step 5: Deploying with Kubernetes
To manage and scale the microservices, we can deploy them using Kubernetes.
Create deployment and service files for the laptop service:
# laptops-deployment. yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: laptops-service
spec:
replicas: 3
selector:
matchLabels:
app: laptops
template:
metadata:
labels:
app: laptops
spec:
containers:
- name: laptops-service
image: laptops-service:latest
ports:
- containerPort: 5000
# laptops-service.yaml
apiVersion: v1
kind: Service
metadata:
name: laptops-service
spec:
selector:
app: laptops
ports:
- protocol: TCP
port: 80
targetPort: 5000
Create deployment and service files for the port service:
# port-deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: port-service
spec:
replicas: 3
selector:
matchLabels:
app: port
template:
metadata:
labels:
app: port
spec:
containers:
- name: port-service
image: port-service:latest
ports:
- containerPort: 5001
# port-service.yaml
apiVersion: v1
kind: Service
metadata:
name: port-service
spec:
selector:
app: port
ports:
- protocol: TCP
port: 80
targetPort: 5001
Deploy the services to Kubernetes:
kubectl apply -f laptops-deployment.yaml
kubectl apply -f laptops-service.yaml
kubectl apply -f port-deployment.yaml
kubectl apply -f port-service.yaml
-
apiVersion: Specifies the Kubernetes API version.
-
kind: Defines the type of resource.
-
metadata: Provides metadata for the resource.
-
spec: Mentions the desired state of the resource.
-
replicas: Shows the number of replicas for the deployment.
-
selector: Matches labels to identify the resources.
-
ports: Shows the charging ports for the service
Building and deploying microservices with Python using frameworks like Flask and FastAPI, containerizing with Docker, and managing with Kubernetes provide reliable Python web applications that are scalable and maintainable in all environments.
Also Read: How to Choose the Right Python Web Development Company?
How Python microservices can help in scalability & system maintenance?
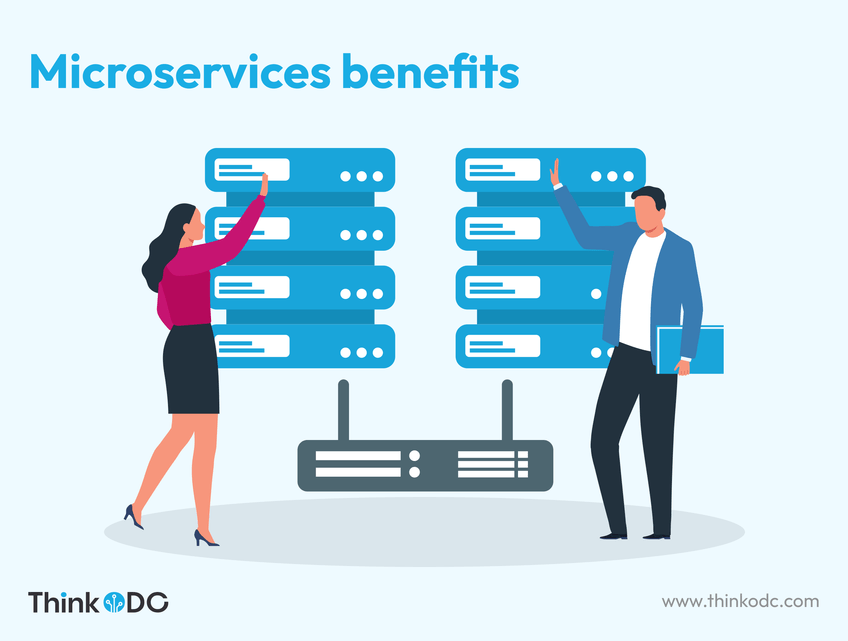
Surely microservice serves a lot of purposes simplifying many complex architectures. But when developers claim that deploying microservices with Python, can help businesses achieve scalable and maintainable systems. That too, paired with efficient resource usage and simplified management of their applications. Then what does it exactly mean? Let’s decode now:
Scalability
- Independent scaling: This benefit marks out that each microservice can be changed or upgraded independently based on its specific load and performance requirements. In simpler terms, it mentions you can allocate more resources to high-demand services without over-provisioning the entire application.
Example: If a microservice that is carrying user authentication faces a sudden spike in traffic due to a large number of users, then it can be scaled up independently of other services like billing or notifications keeping your applications stable & secure even in heavy environments.
- Resource optimization: By isolating services through Python’s microservice architecture businesses can optimize the use of computing resources more effectively. This also guarantees that each service gets the right amount of resources it needs.
Example: Smaller instances can be provided to microservices with low resource requirements, while high-demand services can be allocated to more powerful instances, making your resource management more cost-effective & optimized.
- Flexible technology stack: Microservices utilize different technologies and programming languages for different services, giving an opportunity to use the best tools for each task.
Example: Python language is preferred more for data processing services due to its strong data handling libraries, while other languages are used for high-performance computing tasks. It is all possible due to the flexible features of microservices.
System maintenance
- Simplified updates and deployments: Each microservice can be updated and deployed independently, reducing the complexity and risk associated with deploying an entire application together.
Example: In case of bug fixes or feature updates it can be easily rolled out to individual services without affecting the rest of the system, maintaining a continuous delivery and minimal downtime.
- Enhanced fault isolation: Fault spotted in individual microservices, prevents a single point of failure from bringing down the entire application.
Example: Especially in payment apps if the payment processing service fails, other services like user login or browsing continue to function, keeping a partial system available for the user’s ease.
- Easier debugging and monitoring: Smaller, decoupled services of Python microservices are easier to monitor and debug, allowing precise identification and resolution of issues.
Example: Monitoring tools can track performance and errors in individual services, giving detailed insights into the health of each microservice.
- Improved code maintainability: A modular approach is encouraged by a microservice architecture, making the codebase easier to manage, understand, and maintain.
Example: Developers can focus on specific services, reducing the manual load and sustaining a better organization and documentation of the codebase.
Wrap up
As the world is evolving and the need for secure Python web apps is increasing, adopting architecture patterns that are easily scalable and maintainable without straining available resources is crucial. That’s where microservices architecture built with Python comes in handy! As explored above they can offer immense scalability, development flexibility, and maintainability, making it easier for all scale businesses to manage their complex applications.
At Peerbits our experienced offshore Python developers for hire are updated & skilled in microservices and other latest models. We make sure your infrastructure is secure and maintained by our top 1% talent of IT professionals.
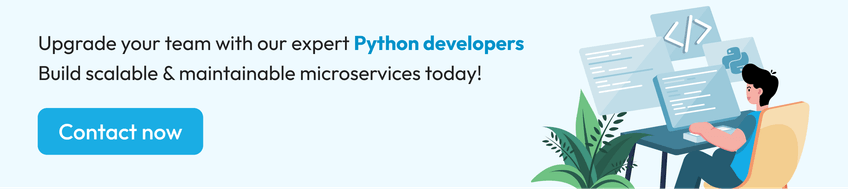